C Programming
Introduction
C is a computer programming language developed in 1972 by Dennis M. Ritchie at the Bell Telephone Laboratories to develop the UNIX Operating System. C is a simple and structure oriented programming language.
C is also called mother Language of all programming Language. It is the most widely use computer programming language, This language is used to develop system software and Operating System. All other programming languages were derived directly or indirectly from C programming concepts. Here we discuss complete C Tutorial in the simple and easy way.
History of C
C language is developed by Mr. Dennis Ritchie in the year 1972 at bell laboratory at the USA, C is a simple and structure Oriented Programming Language.
In the year 1988 C programming language standardized by ANSI (American national standard institute), that version is called ANSI-C. In the year 2000 C programming language standardized by ISO that version is called C-99. All other programming languages were derived directly or indirectly from C programming concepts.
Prerequisites
Before learning C Programming language or this C Tutorial no need of knowledge of any Computer programming language, C is the basis of all high-level programming languages. C is also called mother Language.
Comments in C
Generally, Comments are used to provide the description of the Logic written in the program. Comments are not displayed on the output screen.
When we have used the comments, then that specific part will be ignored by the compiler.
In 'C' language two types of comments are possible
-
Single line comments
-
Multiple line comments
​
Single line comments
Single line comments can be provided by using / /....................
​
Multiple line comments
Multiple line comments can be provided by using /*......................*/
Note: When we are working with the multiple line comments then nested comments are not possible.
​
Rules for Writing Comments
1. The program contains any number of comments at any place.
Example
​
// header files
#include<stdio.h>
#include<conio.h>
void main() {
// variable declaration
int a,b,c;
a=10;
b=20;
c=a+b;
printf("Sum= %d",c);
getch();
}
​
2. Nested Comments are not possible, that means comments within comments.
Example
​
void main()
{
/*
/* comments */
*/
}
​
3. Comments can be splits over more than one line.
Example
​
void main()
{
/* main function body part */
}
​
4. Comments are not case sensitive.
Example
​
void main()
{
/* MAIN Function BODY */
}
​
5. Single line comments start with "//"
Example
​
void main() {
// Single line comment
}
Keywords in C
Keyword is a predefined or reserved word in C library with a fixed meaning and used to perform an internal operation. C Language supports 32 keywords.
Every Keyword exists in lower case latter like auto, break, case, const, continue, int etc.
32 Keywords in C Language
auto double int struct
break else long switch
case enum register typedef
char extern return union
const float short unsigned
continue for signed void
default goto sizeof volatile
do if static while
Types of Constant in C
It is an identifier whose value can not be changed at the execution time of the program. In general, constant can be used to represent as fixed values in a C program. Constants are classified into following types.
If any single character (alphabet or numeric or special symbol) is enclosed between single cotes ' ' known as single character constant.
If the set of characters are enclosed between double cotes " " known as string character constant.
Declare constant
const keyword is used to declare a constant.
Syntax​
const int height = 100;
​
Example​
#include<stdio.h>
#include<conio.h>
void main()
{
const int a=10;
printf("%d",a);
a=20;
// gives error you can't modify const
getch();
}
​
Variable in C Language
Variable is an identifier which holds data or another one variable. It is an identifier whose value can be changed at the execution time of a program. It is used to identify input data in a program.
Syntax:
Syntax
Variable_name = value;
Rules to declare a Variable
To Declare any variable in C language you need to follow rules and regulation of C Language, which is given below;
-
Every variable name should start with alphabets or underscore (_).
-
No spaces are allowed in variable declaration.
-
Except underscore (_) no other special symbol is allowed in the middle of the variable declaration (not allowed -> roll-no, allowed -> roll_no).
-
Maximum length of the variable is 8 characters depend on compiler and operation system.
-
Every variable name always should exist in the left hand side of assignment operator (invalid -> 10=a; valid -> a=10;).
-
No keyword should access variable name (int for <- invalid because for is a keyword).
Note: In a c program variable name always can be used to identify the input or output data.
Variable declarations
This is the process of allocating sufficient memory space for the data in term of the variable.
Syntax
Datatype variable_name;
Example
int a;
If no input values are assigned by the user then the system will give a default value called garbage value.
Garbage value
Garbage value can be any value given by system and that is no way related to correct programs. This is a disadvantage of C programming language and in C programming it can overcome using variable initialization.
Variable initialization
It is the process of allocating sufficient memory space with user-defined values.
Syntax
Datatype nariable_name=value;
Example
int b = 30;
Variable assignment
It is a process of assigning a value to a variable.
Syntax
Variable_Name = value
Example
int a= 20; int b;
Example
b = 25;
// --> direct assigned variable
b = a;
// --> assigned value in term of variable
b = a+15;
// --> assigned value as term of expression
DataType in C Language
Data type is a keyword used to identify the type of data. Data types are used for storing the input of the program into the main memory (RAM) of the computer by allocating sufficient amount of memory space in the main memory of the computer.
In other words, data types are used for representing the input of the user in the main memory (RAM) of the computer.
In general every programming language is containing three categories of data types. They are
-
Fundamental or primitive data types
-
Derived data types
-
User-defined data types
Primitive data types
These are the data types whose variable can hold maximum one value at a time, in C language it can be achieved by int, float, double, char.
Example
int a;
// valid
a = 10,20,30;
// invalid
Derived data types
These data types are derived from the fundamental data type. Variables of derived data type allow us to store multiple values of the same type in one variable but never allows to store multiple values of different types. These are the data type whose variable can hold more than one value of similar type. In C language it can be achieved by an array.
Example
int a[] = {10,20,30};
// valid
int b[] = {100, 'A', "ABC"};
// invalid
User-defined data types
User-defined data types related variables allows us to store multiple values either of the same type or different type or both. This is a data type whose variable can hold more than one value of the dissimilar type, in C language it is achieved by a structure.
Syntax
struct emp
{
int id;
char ename[10];
float sal;
};
In C language, user-defined data types can be developed by using struct, union, enum etc.
Operators
Operator is a special symbol that tells the compiler to perform specific mathematical or logical Operation.
-
Arithmetic Operators
-
Relational Operators
-
Logical Operators
-
Bitwise Operators
-
Assignment Operators
-
Ternary or Conditional Operators
Arithmetic Operators
Given table shows all the Arithmetic operator supported by C Language. Let's suppose variable A hold 8 and B hold 3.
OperatorExample (int A=8, B=3) Result
+ A+B 11
- A-B 5
* A*B 24
/ A/B 2
% A%4 0
Relational Operators
Which can be used to check the Condition, it always returns true or false. Let's suppose variable Ahold 8 and B hold 3.
OperatorsExample (int A=8, B=3) Result
< A<B False
<= A<=10 True
> A>B True
>= A<=B False
== A== B False
!= A!=(-4) True
Logical Operator
Which can be used to combine more than one Condition?. Suppose you want to combine two conditions A<B and B>C, then you need to use Logical Operator like (A<B) && (B>C). Here &&is Logical Operator.
OperatorExample (int A=8, B=3, C=-10) Result
&& (A<B) && (B>C) False
|| (B!=-C) || (A==B) True
! !(B<=-A) True
A truth table of Logical Operator
C1 C2 C1 && C2 C1 || C2 !C1 !C2
T T T T F F
T F F T F T
F T F T T F
F F F F T T
Assignment operators
Which can be used to assign a value to a variable. Let's suppose variable A holds 8 and B hold 3.
OperatorExample (int A=8, B=3) Result
+= A+=B or A=A+B 11
-= A-=3 or A=A+3 5
*= A*=7 or A=A*7 56
/= A/=B or A=A/B 2
%= A%=5 or A=A%5 3
= a=b Value of b will be assigned to a
First Program
Programming in C language is very simple and it is easy to learn here I will show you how to write your first program. For writing C program you need Turbo C Editor. First, you open TC and write code.
Example
#include<stdio.h>
#include<conio.h>
void main()
{
printf("This is my first program");
getch();
}
Output
This is my first program
-
After writing complete code save the program using F2
-
Save your code with .c extension for example: hello.c
-
After saving the code you need to compile your code using alt+f9
-
Finally, Run the program using clt+f9
Save C program
Save any C program using .c Extension with the file name. For example, your program name is sum, then it save with sum.c.
Syntax
filename.c
Compile and Run C Program
for compile any C program you just press alt+f9, after compilation of your c program you press clt+f9 for running your C program.
Syntax
Compile -> alt+f9 Run -> clt+f9
sizeof operator
The sizeof operator is used to calculate the size of data type or variables. This operator returns the size of its variable in bytes.
For example, sizeof(a), where a is an integer, will return 4.
Syntax
sizeof(variable)
Example
#include<stdio.h>
#include<conio.h>
void main() {
int a;
float b;
double c;
char d;
printf("Size of Integer: %d bytes\n",sizeof(a));
printf("Size of float: %d bytes\n",sizeof(b));
printf("Size of double: %d bytes\n",sizeof(c));
printf("Size of character: %d byte\n",sizeof(d));
getch();
}
Output
Size of Integer: 2
Size of float: 4
Size of double: 8
Size of character: 1
main() function in C
main() function is the entry point of any C program. It is the point at which execution of the program is started. When a C program is executed, the execution control goes directly to the main() function. Every C program has a main() function.
Syntax
void main() { ......... ......... }
In above syntax;
-
void: is a keyword in C language, void means nothing, whenever we use void as a function return type then that function nothing return. here main() function no return any value.
-
In place of void, we can also use int return type of main() function, at that time main() return integer type value.
-
main: is a name of the function which is predefined function in C library.
Simple example of main()
Example
#include<stdio.h>
void main()
{
printf("This is main function");
}
Output
This is main function
Printf()
Printf is a predefined function in "stdio.h" header file, by using this function, we can print the data or user-defined message on console or monitor. While working with printf(), it can take any number of arguments but the first argument must be within the double cotes (" ") and every argument should separate with comma (,) Within the double cotes, whatever we pass, it prints same, if any format specifies are there, then that copy the type of value. The scientific name of the monitor is called console.
Syntax
printf("user defined message");
Syntax
prinf("Format specifers",value1,value2,..);
Example of printf function
int a=10;
double d=13.4;
printf("%f%d",d,a);
scanf()
scanf() is a predefined function in "stdio.h" header file. It can be used to read the input value from the keyword.
Syntax
scanf("format specifiers",&value1,&value2,.....);
Example of scanf function
int a;
float b;
scanf("%d%f",&a,&b);
In the above syntax format specifier is a special character in the C language used to specify the data type of value.
Format specifier:
Format specifier Type of value
%d Integer
%f Float
%lf Double
%c Single character
%s String
%u Unsigned int
%ld Long int
%lf Long double
The address list represents the address of variables in which the value will be stored.
Example
int a;
float b;
canf("%d%f",&a,&b);
In the above example scanf() is able to read two input values (both int and float value) and those are stored in a and b variable respectively.
Syntax
double d=17.8; char c; long int l; scanf("%c%lf%ld",&c&d&l);
Example of printf() and scanf()
#include <stdio.h>
#include <conio.h>
void main(); {
int a;
float b;
clrscr();
printf("Enter any two numbers: ");
scanf("%d %f",&a,&b);
printf("%d %f",a,b); getch();
}
Output
Enter any two numbers: 10 3.5
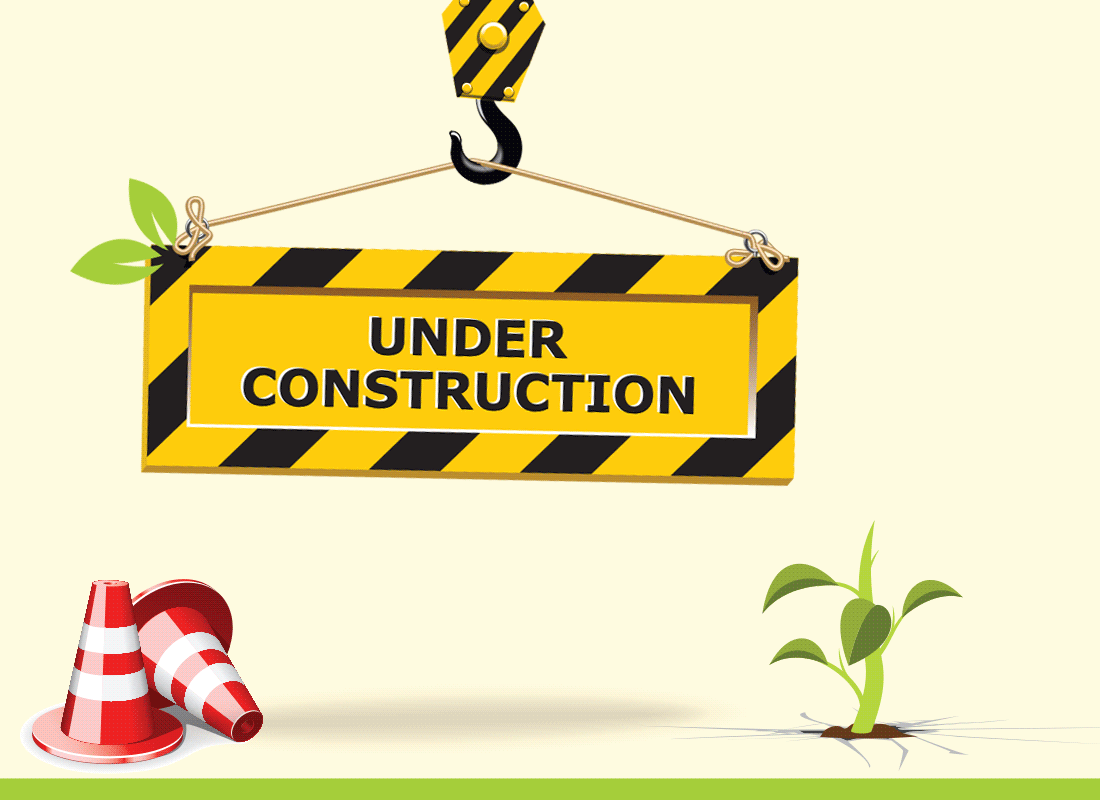